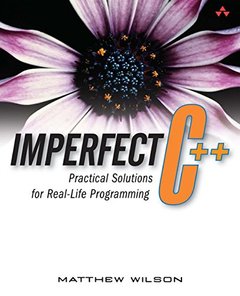
Imperfect C++ : Practical Solutions for Real-Life Programming
內容描述
Description:
Imperfect C++
C++, although a marvelous language, isn't
perfect. Matthew Wilson has been working with it for over a decade, and during
that time he has found inherent limitations that require skillful workarounds.
In this book, he doesn't just tell you what's wrong with C++, but offers
practical techniques and tools for writing code that's more robust, flexible,
efficient, and maintainable. He shows you how to tame C++'s complexity, cut
through its vast array of paradigms, take back control over your code—and get
far better results.
If you're a long-time C++ developer, this book
will help you see your programming challenges in new ways—and illuminate
powerful techniques you may never have tried. If you're newer to C++, you'll
learn principles that will make you more effective in all of your projects.
Along the way, you'll learn how to:
Overcome deficiencies in C++'s type
system
Enforce software design through constraints,
contracts, and assertions
Handle behavior ignored by the standard—
including issues related to dynamic libraries, static objects, and
threading
Achieve binary compatibility between dynamically
loading components
Understand the costs and disadvantages of
implicit conversions—and the alternatives
Increase compatibility with diverse compilers,
libraries, and operating environments
Help your compiler detect more errors and work
more effectively
Understand the aspects of style that impact
reliability
Apply the Resource Acquisition Is Initialization
mechanism to a wide variety of problem domains
Manage the sometimes arcane relationship between
arrays and pointers
Use template programming to improve flexibility
and robustness
Extend C++: including fast string concatenation,
a true NULL-pointer, flexible memory buffers, Properties, multidimensional
arrays, and Ranges
The CD-ROM contains a valuable variety of C++
compilers, libraries, test programs, tools, and utilities, as well as the
author's related journal articles. New and updated imperfections, along with
software libraries and example code are available online at http://imperfectcplusplus.com.
Table of
Contents:
I. FUNDAMENTALS.
- Enforcing Design: Constraints, Contracts, and
Assertions.
Eggs and Ham.
Compile-Time Contracts: Constraints.
Runtime Contracts: Preconditions,
Postconditions, and Invariants.
Assertions.
- Object Lifetime.
The Object Life Cycle.
Controlling Your Clients.
MILs and Boon. - Resource Encapsulation.
A Taxonomy of Resource Encapsulation.
POD Types.
Wrapper Proxies.
RRID Types.
RAII Types.
RAII Coda.
- Data Encapsulation and Value Types.
A Taxonomy of Data Encapsulation.
Value Types and Entity Types.
A Taxonomy of Value Types.
Open Types.
Encapsulated Types.
Value Types.
Arithmetic Value Types.
Value Types Coda.
Encapsulation Coda.
- Object Access Models.
Vouched Lifetimes.
Copied for Caller.
Given to Caller.
Shared Objects. - Scoping Classes.
Value.
State.
APIs and Services.
Language Features.
II. SURVIVING THE REAL WORLD. - ABI.
Sharing Code.
C ABI Requirements.
C++ ABI Requirements.
I Can C Clearly Now. - Objects Across Borders.
Mostly Portable vtables?
Portable vtables.
ABI/OAB Coda. - Dynamic Libraries.
Calling Functions Explicitly.
Indentity: Link Units and Link Space.
Lifetime.
Versioning.
Resource Ownership.
Dynamic Libraries: Coda.
- Threading.
Synchronizing Integer Access.
Synchronizing Block Access: Critical
Regions.
Atomic Integer Performance.
Multithreading Extensions.
Thread Specific Storage.
- Statics.
Nonlocal Static Objects: Globals.
Singletons.
Function-Local Static Objects.
Static Members.
Statics Coda.
- Optimization.
Inline Functions.
Return Value Optimization.
Empty Base Optimization.
Empty Derived Optimization.
Preventing Optimization.
III. LANGUAGE CONCERNS. - Fundamental Types.
May I Have a byte?
Fixed-Sized Integer Types.
Large Integer Types.
Dangerous Types. - Arrays and Pointers.
Don't Repeat Yourself.
Arrays Decay into Pointers.
dimensionof().
Cannot Pass Arrays to Functions.
Arrays Are Always Passed by Address.
Arrays of Inherited Types.
Cannot Have Multidimensional Arrays.
- Values.
NULL-The Keyword That Wasn't.
Down to Zero.
Bending the Truth.
Literals.
Constants.
- Keywords.
interface.
temporary.
owner.
explicit(_cast).
unique.
final.
Unsupported Keywords. - Syntax.
Class Layout.
Conditional Expressions.
for.
Variable Notation. - Typedefs.
Pointer typedefs.
What's in a Definition?
Aliases.
True Typedefs.
The Good, the Bad, and the Ugly.
IV. COGNIZANT CONVERSIONS.
- Casts.
Implicit Conversion.
Casting in C++.
The Case for C Casts.
Casts on Steroids.
explicit_cast.
literal_cast.
union_cast.
comstl::interface_cast.
boost::polymorphic_cast.
Casts: Coda. - Shims.
Embracing Change and Enhancing
Flexibility.
Attribute Shims.
Logical Shims.
Control Shims.
Conversion Shims.
Composite Shim Concepts.
Namespaces and Koenig Lookup.
Why Not Traits?
Structural Conformance.
Breaking Up the Monolith.
Shims: Coda.
- Veneers.
Lightweight RAII.
Binding Data and Operations.
Rubbing Up to Concepts.
Veneers: Coda.
- Bolt-ins.
Adding Functionality.
Skin Selection.
Nonvirtual Overriding.
Leveraging Scope.
Simulated Compile-Time Polymorphism:
Reverse Bolt-ins.
Parameterized Polymorphic Packaging.
Bolt-ins: Coda.
- Template Constructors.
Hidden Costs.
Dangling References.
Template Constructor Specialization.
Argument Proxies.
Argument Targeting.
Template Constructors: Coda.
V. OPERATORS.
- operator bool().
operator int() const.
operator void () const.
operator bool() const.
operator !()-not!.
operator boolean const () const.
operator int boolean::() const.
Operating in the Real World.
operator!.
- Fast, Non-intrusive String Concatenation.
fast_string_concatenator<>.
Performance.
Working with Other String Classes.
Concatenation Seeding.
Pathological Bracing.
Standardization.
- What's Your Address?
Can't Get the Real Address.
What Actions Are Carried Out during
Conversion?
What Do We Return?
What's Your Address: Coda. - Subscript Operators.
Pointer Conversion versus Subscript
Operators.
Handling Errors.
Return Value. - Increment Operators.
Missing Postfix Operators.
Efficiency. - Arithmetic Types.
Class Definition.
Default Construction.
Initialization (Value Construction).
Copy Construction.
Assignment.
Arithmetic Operators.
Comparison Operators.
Accessing the Value.
sinteger64.
Truncations, Promotions, and Tests.
Arithmetic Types: Coda.
- Short-circuit!
VI. EXTENDING C++. - Return Value Lifetime.
A Taxonomy of Return Value Lifetime
Gotchas.
Why Return-by-Reference?
Solution 1-integer_to_string<>.
Solution 2-TSS.
Solution 3-Extending RVL.
Solution 4-Static Array Size
Determination.
Solution 5-Conversion Shims.
Performance.
RVL: The Big Win for Garbage
Collection.
Potential Applications.
Return Value Lifetime: Coda.
- Memory.
A Taxonomy of Memory.
The Best of Both Worlds.
Allocators.
Memory: Coda. - Multidimensional Arrays.
Facilitating Subscript Syntax.
Sized at Run Time.
Sized at Compile Time.
Block Access.
Performance.
Multidimensional Arrays: Coda.
- Functors and Ranges.
Syntactic Clutter.
for_all() ?
Local Functors.
Ranges.
Functors and Ranges: Coda. - Properties.
Compiler Extensions.
Implementation Options.
Field Properties.
Method Properties.
Static Properties.
Virtual Properties.
Property Uses.
Properties: Coda.
Appendix A. Compilers and Libraries.
Compilers.
Libraries.
Other Resources.
Appendix B. "Watch That Hubris!"
Operator Overload.
DRY Rued Yesterday.
Paranoid Programming.
To Insanity, and Beyond!
Appendix C. Arturius.
Appendix D. The CD.
Epilogue.
Bibliography.
Index.